Copied documentation by Bro. Three
Warning
- Please back up your source code first. I am not responsible for any file loss.
- There is no guarantee that this script will keep on working in the future, especially if Steam decides to do a major update to their system.
Setup
- Download the OrangeGreenworks plugin. This plugin lets you use Greenworks to integrate your game with the Steamworks API.
- Enable the plugin in your project.
- Download the latest version of Greenworks (as of 12/1/2024, the latest version is v0.15).
- If you use Electron, go for “Electron”, otherwise, go for the one with the label “nw”.
- You don’t have to download everything, only the OS you need. 95% of the time, you will be using Win64.
- After downloading the .zip file, you will find a folder called “lib” and a .js file. Put them both in your game’s source code’s main folder. (The folder with the index.html file)
- You only need one copy of the .js file as it is the same throughout different OS.
- Next, download the Steamworks SDK for which your Greenworks “lib” is compiled.
- The Greenworks release page should indicate the version you need. For example, Lukewarm Massacre uses Greenworks v0.15, which is compiled for Steamworks SDK v1.58.
- The Steamworks SDK can be downloaded from the Steamworks website, just log in to your Steamworks account.
- Copy (and rename if necessary) the following files from the SDK to the “lib” folder. You can ignore any OS that you will not be using.
- Follow the table below, yellow cells indicate that you must change the file’s name:
- Go to RPG Maker MV and export (deploy) your game to any OS you want.
- If you have upgraded your nw.js in the local files of RPG Maker MV so that the nw.js version is compatible with the Greenworks version that you are using, you can skip Steps 9 to 11 (click here to see how you can upgrade your NW.js). If not, or if you are unsure, or just to be safe, continue to Step 9.
- Go to the “www” folder and make sure the files you copied from Step 6 are present as well as the .js file in the root folder. (If not, do not proceed. Try repeating Steps 4 to 7.)
- If the files are present, go back outside of the “www” folder. Leave the “www” folder and the “package.json” file as is. Delete everything else.
- Now, download the “nw.js” file here. Check the Greenworks page to see which version you need. For example, Greenworks v0.15 requires nw.js v0.82. Do not download the wrong version. Extract and place all the files where you removed the older files.
- There will be two versions of the file.
- The SDK version allows you to access the console by pressing F12, good for debugging and testing purposes.
- In the Normal version, there is no way to access the console, it’s better for final releases.
- You can go straight for the Normal version if you are confident that you will not run into any errors, or if you can solve any errors. Otherwise, you should download the SDK version first.
- Now, create a file and name it “steam_appid.txt” in the same folder where you deleted the files (the same place where the “www” folder is present). In the .txt file, simply put in your game’s ID, save the file, and close it.
- In newer versions of Greenworks, you may get an error saying:
“required value ‘name’ is missing”
To fix this, open the package.json with a note editor (something like Notepad or Visual Studio). Change the value of “name” to anything else other than “”.
- At this point, when you run the “nw.exe” file, everything should be working as is and you will see Steam indicating that you are playing the game. If it is then everything is working, congratulations! If not, something must’ve gone wrong.
- If you need to debug the game, go find the “OrangeGreenworks.js” in the exported files. Edit Line 121 so that it says “$.greenworks.init();” instead of “$.greenworks.initAPI();”.
If somehow that piece of code is not at Line 121, use Ctrl+F to find it.
After that, run the “nw.exe” file and press F12, you should be able to get more information on what went wrong.
Possible issues:- Missing Steamworks SDK files, repeat Step 6.
- Steamworks/Greenworks failed to initialise, if it’s caused by the absence of steam_appid.txt, repeat Step 12.
From | To |
---|---|
sdk/redistributable_bin/steam_api.dll |
game/lib/steam_api.dll |
sdk/redistributable_bin/steam_api.lib |
game/lib/steam_api.lib |
sdk/redistributable_bin/win64/steam_api64.dll |
game/lib/steam_api64.dll |
sdk/redistributable_bin/win64/steam_api64.lib |
game/lib/steam_api64.lib |
sdk/redistributable_bin/osx32/libsteam_api.dylib |
game/lib/libsteam_api.dylib |
sdk/redistributable_bin/linux32/libsteam_api.so |
game/lib/libsteam_api.so |
sdk/redistributable_bin/linux64/libsteam_api.so |
game/lib/libsteam_api64.so |
sdk/public/steam/lib/win32/sdkencryptedappticket.dll |
game/lib/sdkencryptedappticket.dll |
sdk/public/steam/lib/win32/sdkencryptedappticket.lib |
game/lib/sdkencryptedappticket.lib |
sdk/public/steam/lib/win64/sdkencryptedappticket64.dll |
game/lib/sdkencryptedappticket64.dll |
sdk/public/steam/lib/win64/sdkencryptedappticket64.lib |
game/lib/sdkencryptedappticket64.lib |
sdk/public/steam/lib/osx32/libsdkencryptedappticket.dylib |
game/lib/libsdkencryptedappticket.dylib |
sdk/public/steam/lib/linux32/libsdkencryptedappticket.so |
game/lib/libsdkencryptedappticket.so |
sdk/public/steam/lib/linux64/libsdkencryptedappticket.so |
game/lib/libsdkencryptedappticket64.so |
Commands
Information
To use these commands, click on the “Script…” (NOT “Plugin Command…”).
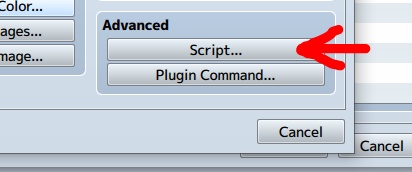
OrangeGreenworks.getScreenName();
This script call will return the Player’s Steam Screen Name. While playtesting, it will return “Play Test” instead.
OrangeGreenworks.getUILanguage();
This command will return the player’s Steam UI language. When playtesting, it will return “English” instead.
OrangeGreenworks.getGameLanguage();
This command will return the player’s chosen language in the game settings. When playtesting, it will return “English” instead.
OrangeGreenworks.getNumberOfAchievements();
This command will return the number of achievements the game has.
To store any of the information, use this script:
$gameVariables.setValue(VARIABLE_ID, INFORMATION);
This command will set the information to a variable, all you need to do is change VARIABLE_ID to the number of the variable that you want to store the information in and the INFORMATION to one of the commands above.
For example:
$gameVariables.setValue(17, OrangeGreenworks.getScreenName());
This will store the player’s Steam screen name to Variable #17 (accessible via \V[17])
Achievements
OrangeGreenworks.activateAchievement('ACHIEVEMENT_API_NAME');
This command will activate an achievement. While playtesting, it will only display a message on the console indicating that the achievement was activated. Replace ACHIEVEMENT_API_NAME with the achievement’s API name, NOT the achievement’s name.

$gameSwitches.setValue(SWITCH_ID, OrangeGreenworks.getAchievement('ACHIEVEMENT_API_NAME'));
This command will check if the player already had the achievement called ACHIEVEMENT_API_NAME and change its value accordingly. Replace SWITCH_ID with the switch’s number ID. Replace ACHIEVEMENT_API_NAME with the achievement’s API name.
OrangeGreenworks.clearAchievement('ACHIEVEMENT_API_NAME');
This command will remove the achievement from the player. Useful for when you are testing the game, but probably won’t be needing it for the actual game… well… unless you’re making a troll game like Shapeshifting Adventure 3.
Others
These commands are available on the Greenworks plugin page:
- activateGameOverlay
- isGameOverlayEnabled
- activateGameOverlayToWebPage
- isSubscribedApp
- getDLCCount
- isDLCInstalled
- installDLC
- uninstallDLC
- getStatInt
- getStatFloat
- setStat
- storeStats
Contact
Bro. Three (no guarantees)Hudell
References
Lehnen, P. H. (2016, March 27). [RPG Maker] Orange Greenworks. Hudell Tales. Retrieved January 12, 2024, from https://web.archive.org/web/20190701082834/http://hudell.com/blog/orangegreenworks/
Back